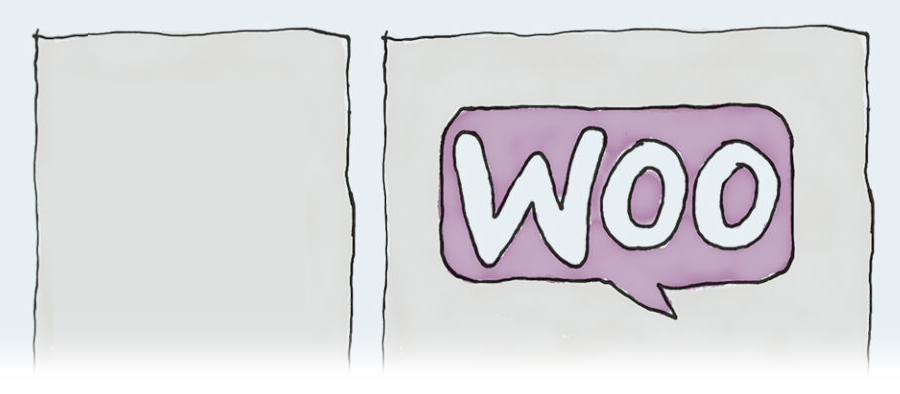
Custom sidebar in Woocommerce without plugins
septembrie 2, 2022 by
Andy
This is a fully custom approach to add a custom sidebar in your Woocommerce template in pure PHP code, no plugins or other stuff like that.
So first, we need to register a new sidebar, which is done in our theme. Usually, the way is highly recommended, you should have a child theme in your WordPress website, which (as most people know) allows your own customisations without impeding the main theme code. The biggest advantage is obviously that when the main theme is updated, you don’t lose any of your custom code.
Based on that, to add a new sidebar we will add a bit of code in our functions.php file, which should live in your child theme directory.
//We need a custom sidebar only for the Shop pages
function my_theme_widgets_init() {
register_sidebar( array(
'name' => __( 'Shop Sidebar', 'my_theme' ),
'id' => 'sidebar-shop',
'description' => __( 'The main sidebar appears on the left of the Shop page', 'my_theme' ),
'before_widget' => '<aside id="%1$s" class="widget %2$s">',
'after_widget' => '</aside>',
'before_title' => '<h3 class="widget-title">',
'after_title' => '</h3>',
) );
}
add_action( 'widgets_init', 'my_theme_widgets_init' );
In there, replace “my_theme” with your theme name/slug.
After that, when you go to you WP admin, under the Customize > Widgets area, you should already see your new sidebar listed with the above name. You can add a few widgets, just to have something populated.
Adding the sidebar to the Woocommerce template
Now, to add it to the actual Woocommerce template it’s a bit more complicated, as each theme has its own compatibility with Woo. Some of them already have implemented some sidebar support, some not.
In this case we will exclude all of that and will consider the main theme doesn’t have any Woo sidebar support.
So the way Woo templating works is that you can overwrite the templating functionality by copying the default Woo template files from the /plugins/woocommerce folder to your own child theme, in the {your_childe_theme}/woocommerce folder
Now, the exact template file that deals with the main products listing page is \wp-content\themes\{your_childe_theme}\woocommerce\archive-product.php
In there the code adds some HTML wraper tags and then loops through the Woo products a lists them in their specific tags as well.
In order to add the sidebar, I used a great helper – Bootstrap – which was already included in the main theme that the website happened to use – Generatepress – but if your theme doesn’t have it, it can be easily added to your WordPress as an external library.
Now, to take advantage of the BS, we use its classes that organize the layout into 2 columns, one smaller to the left (the sidebar) and the other wider to the right (the products). The classes are “col-2” and “col-10” and the code we add, just after the “</header>” is:
<div class="row">
<div class="col-2 border-right" style="height:98%;">
<?php
//get_sidebar( 'sidebar-shop' );
//dynamic_sidebar( 'smartslider_area_1' );
if ( is_active_sidebar( 'sidebar-shop' ) ) {
//echo '<ul id="sidebar">';
dynamic_sidebar( 'sidebar-shop' );
//echo '</ul>';
}
echo '</div><div class="col-10">';
if ( woocommerce_product_loop() ) {
/**
* Hook: woocommerce_before_shop_loop.
*
* @hooked woocommerce_output_all_notices - 10
* @hooked woocommerce_result_count - 20
* @hooked woocommerce_catalog_ordering - 30
*/
do_action( 'woocommerce_before_shop_loop' );
woocommerce_product_loop_start();
if ( wc_get_loop_prop( 'total' ) ) {
while ( have_posts() ) {
the_post();
/**
* Hook: woocommerce_shop_loop.
*/
do_action( 'woocommerce_shop_loop' );
wc_get_template_part( 'content', 'product' );
}
}
woocommerce_product_loop_end();
//close divs 'col-10' and 'row'
echo '</div></div>';
Note 1: that at the end, after “woocommerce_product_loop_end();” we need to close the Bootstrap columns tags with ‘</div></div>’
Note 2: You can style anything in there with your own child theme custom CSS that again most of you know how to use. If not, here is a guide.
The template we altered is valid for the main product listing, but when you click on a product you go to an individual product page, which has another template. That one needs to be customised as well with the sidebar. This is its corresponding template that needs to be copied into your child theme: wp-content\themes\{your_theme}\woocommerce\single-product.php
Now when you go to your site main shop page (usually www.yoursite.com/shop/) you should see your new sidebar with whatever widgets contact you chose in the admin. You can continue to add more widgets and see them refresh instantly.
Happy coding and let me know if this helped or ask any other questions.